Content
Brief backgroundStarting LARA server
Authentication system
API system
Serialization system
Packet system
Lara Framework
Serialization system
Lara framework is equiped with high performance binary serialization system that supports serialization of the most common types.
To use the serialization system, the models to be serilized must be inheriting from the abstract type "Lara.Info".
The types that inherit from Info class can be converted to byte array and easily transferred over network or saved to disk as a binary file.
So, let's declare a simple user model:
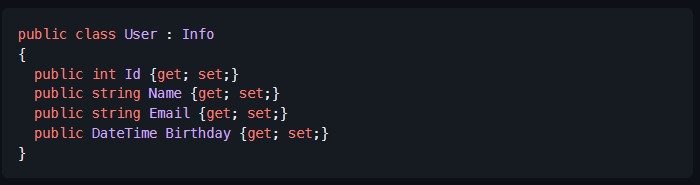
let's instantiate our user with values:
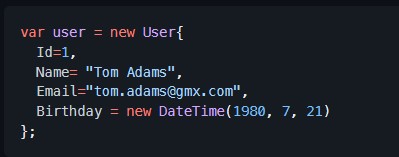
Example of simple serialization:

It is also possible to use a password encrypted Serialization.
Through utilisation of AES256 algorithm a password with maximum length of 32 charachter can be used.
An example looks like this:

Deserialization
The received byte array can be easily deserialized on the other network side, given that the user class is also declared there.
When using deserialization we must know beforehand the type of the original Info object and pass it as a generic argument.
This generic argument must always be of type Info(i.e. a class that is inheriting the abstract Info class).
If we pass a wrong type, null will be returned.
An example of simple deserialization:
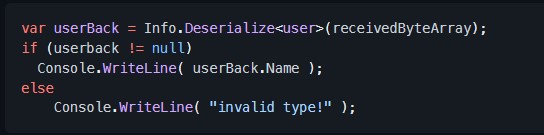
For deserializing password encrypted binary array, we need to enter the correct password, otherwise null will be returned.
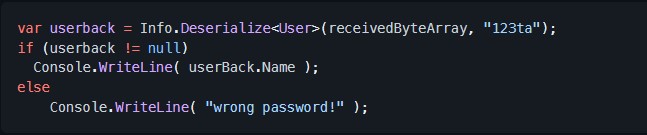
any inconsistency will result in "RequestStatus.Unknown_type" upon calling "Request" method!
It is a good practice to have all Info objects under "Models" namespace and in a directory named "Models" in your project map, like the example here:
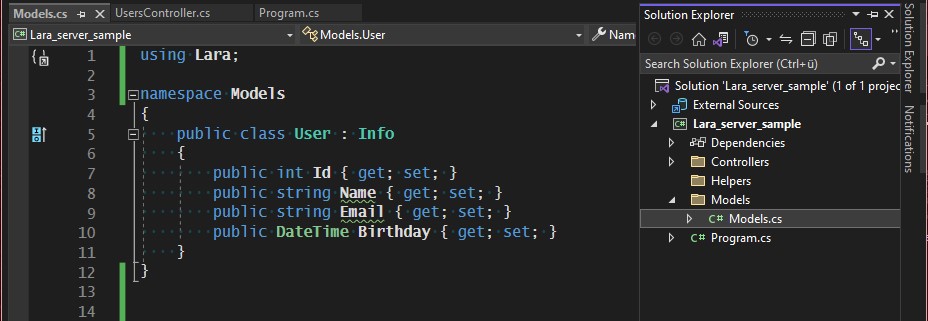
Send Info objects through API call
Info objects can be easily transferd between server and client using Lara API system.
In the following example, we will walk through sending a list of randomly generated User objects.
First, we create a random name generator class to fill the "Name" property of our user class:
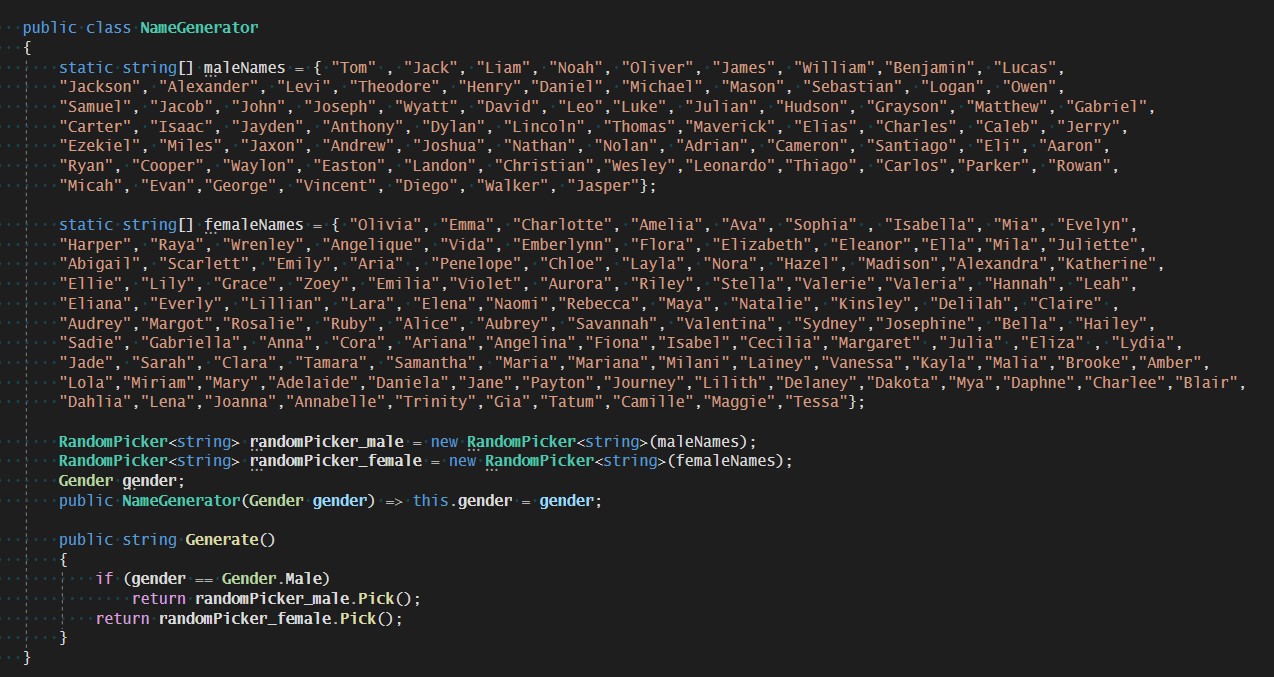
Then, we crete a random Email generator class to fill the "Email" property of our user class:
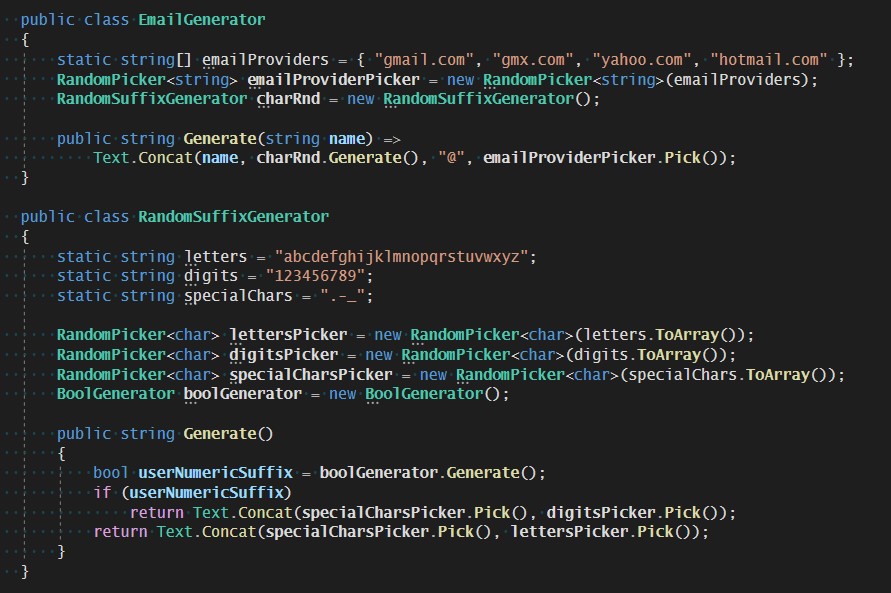
Then, we crete a random user generator class to generate random user objects:
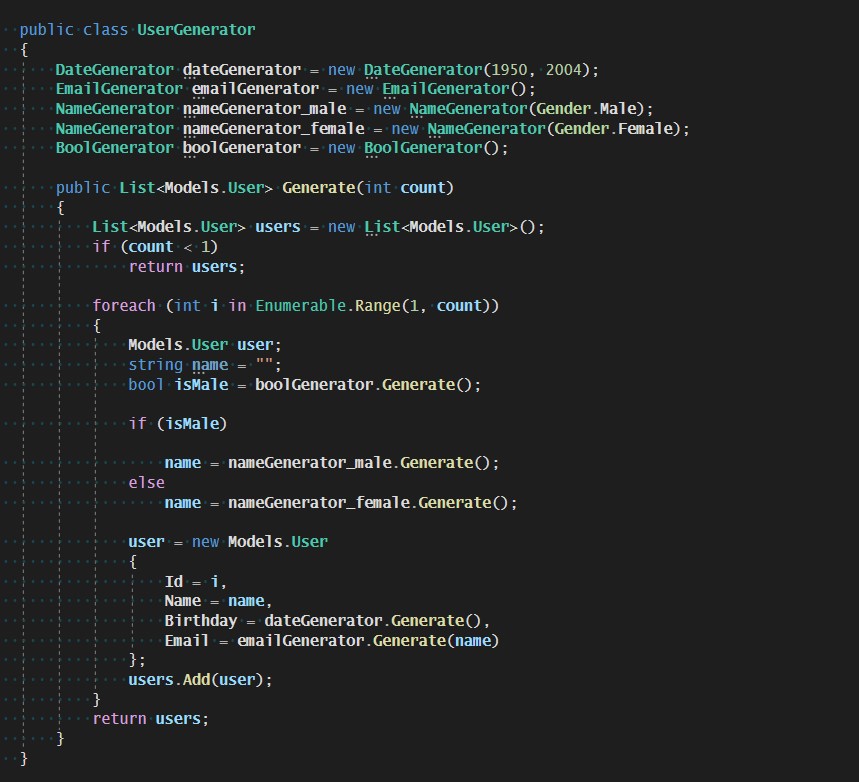
Now, we create the controller, that holds our new API. this API will be called from client through Lara API system.
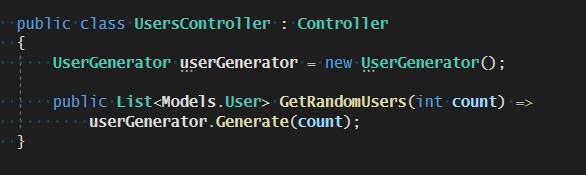
Let's not forget to add the controller to Server through dependency injection!
If we forget to do that "RequestStatus.Controller_invalid" will be returned on the client side when calling Request method!

Now that we finished the server part, let's go to the client and call some users from server!
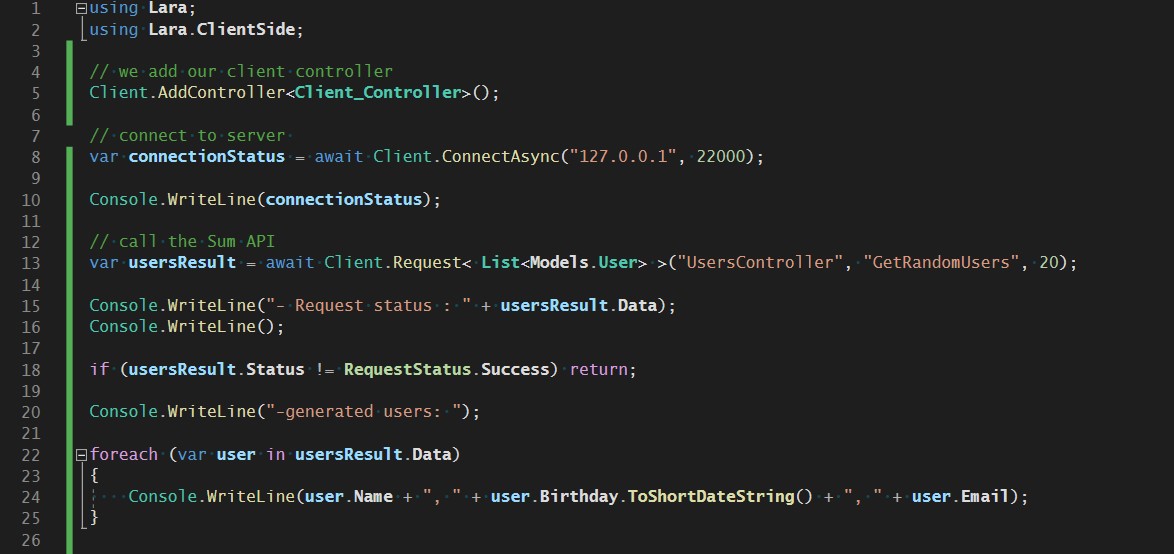
And as you see, here is the requsted list of users:
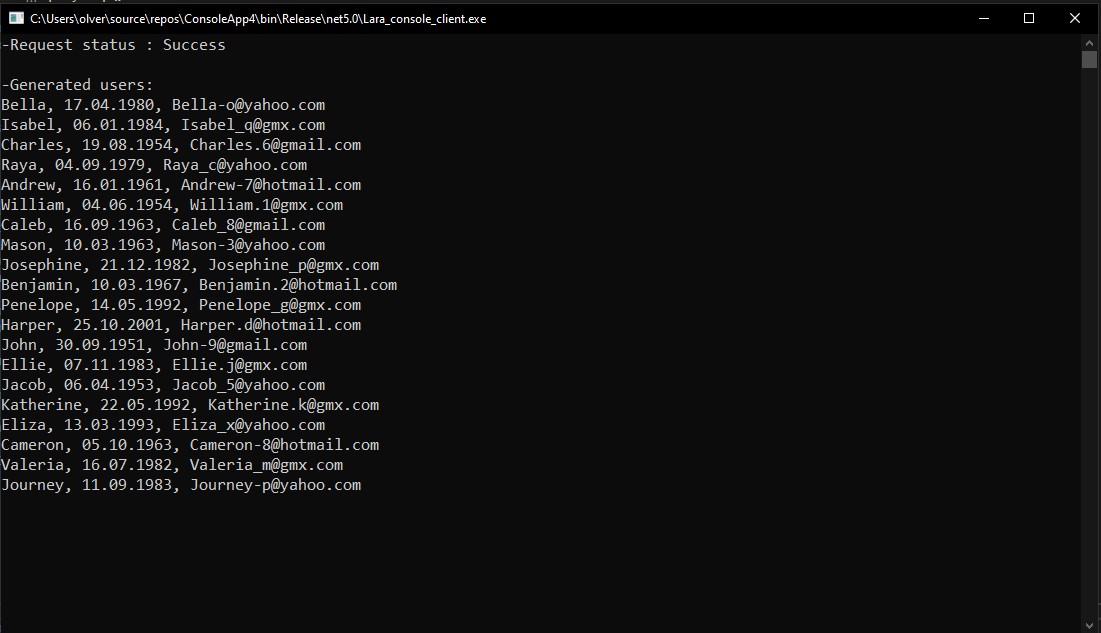
Back to API system
Go to Packet system