Content
Brief backgroundStarting LARA server
Authentication system
API system
Serialization system
Packet system
Lara Framework
Lara API system
Lara framework is equiped with a robust API call system, that enables developers to create server- and client-side APIs and call them asyncronously.
To handle requests a controller must be created and added to server or client through dipendency injection.
Server-side APIs
In Lara framework it is possible to add server-side as well as client-side APIs.
In order to use either of these, we must first create a controller that holds our APIs. On client-side the client controller is "Lara.ClientSide.Controller, on server-side "Lara.ServerSide.Controller".
Now, let's create a sample API-Controller on server by inheriting from Lara.ServerSide.Controller.
We will name this controller "Math_Controller", then we will add 2 methods "Sum" and "RandomInt".
If every thing is correct, we should be able to call these methods from client-side using "Client.Request" method.
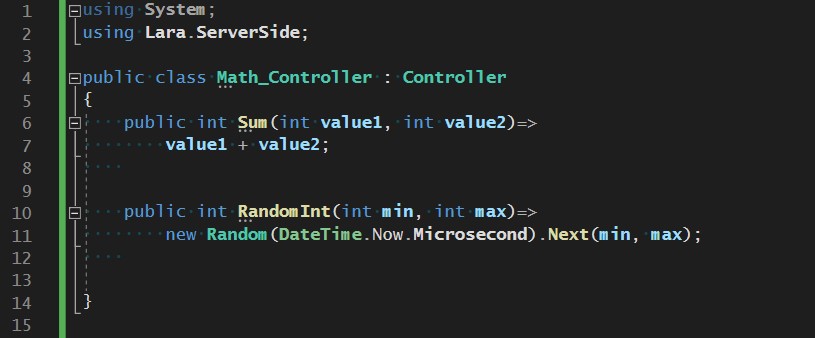
Now, let's add our "Math_Controller" to the server through dependency injection:
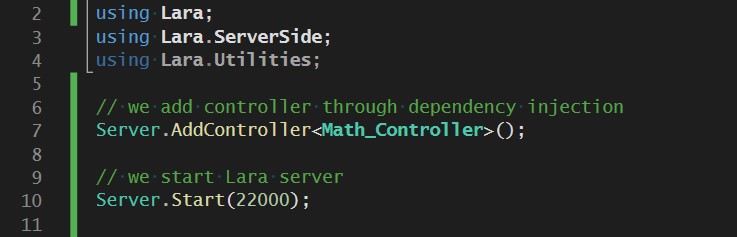
Calling server-APIs from client-side
The decleration of the method to request server-side APIs on client looks like this:

This method will return the generic AsyncResponse object, which encapsulates the reponse status as well as the data returned from the API call.
When AsyncResponse.Status is equal to RequestStatus.Success the AsyncResponse.Data will contain the requested data from the API call, otherwise, default(T) will be returned.
Parameters:
-controllerName : The controller, that holds our method, that we want to call( The controller, that we created was "Math_Controller").
-actionName : The method, inside the controller , that we want to call(We created 2 method "Sum" and "RandomInt").
-arguments : The arguments, that our method takes.
Notice: "Request" method declares the arguments as an object array, so an explicit casting can be required to prevent
ambiguity, for example whole numeric values like uint, short, ushort and byte must be explicitly casted to thier type,
that is declared in the API method. By failing to do so, these values will be considered by the combiler as integer literals and RequestStatus.Arguments_invalid
will be returned in the AsyncResponse.Status.
Now, let's call our API using this method from client-side:
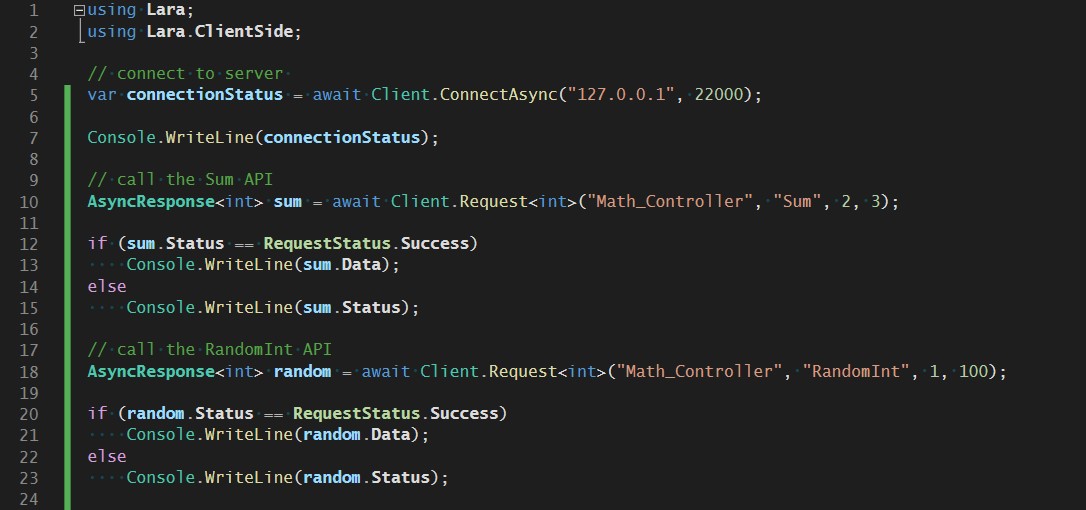
Console output:
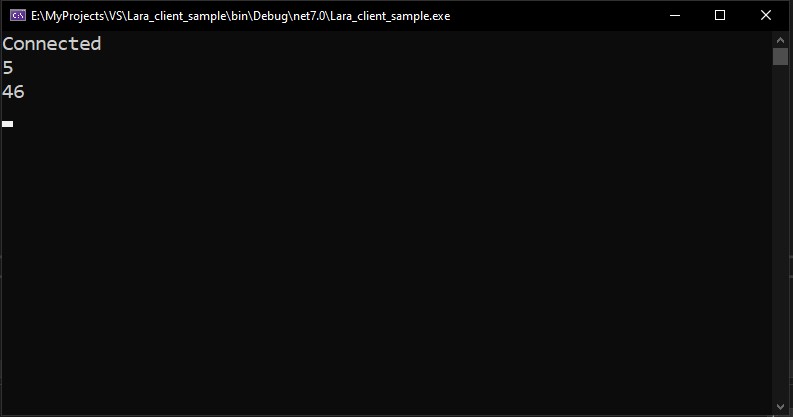
Client-side APIs
To create a client-side API, we must first create a client controller(a class that inherts from Lara.ClientSide.Controller).
Now, let's create a sample API-Controller on client-side:
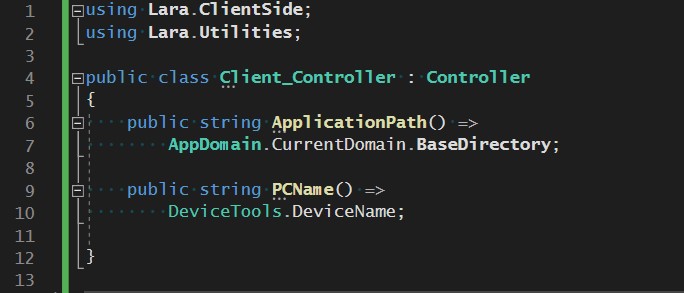
Now, let's add our controller to the client through dependency injection:
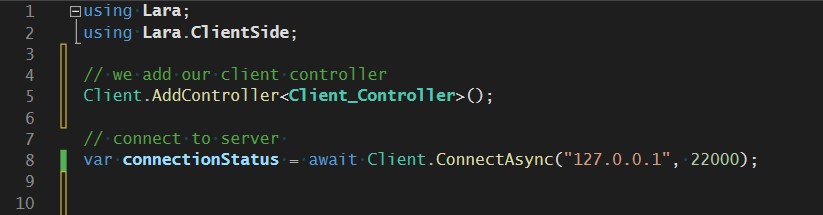
Calling client-APIs from server-side
The decleration of the method to request client-side APIs from server looks like this:

Now, let's call our API using this method on server:
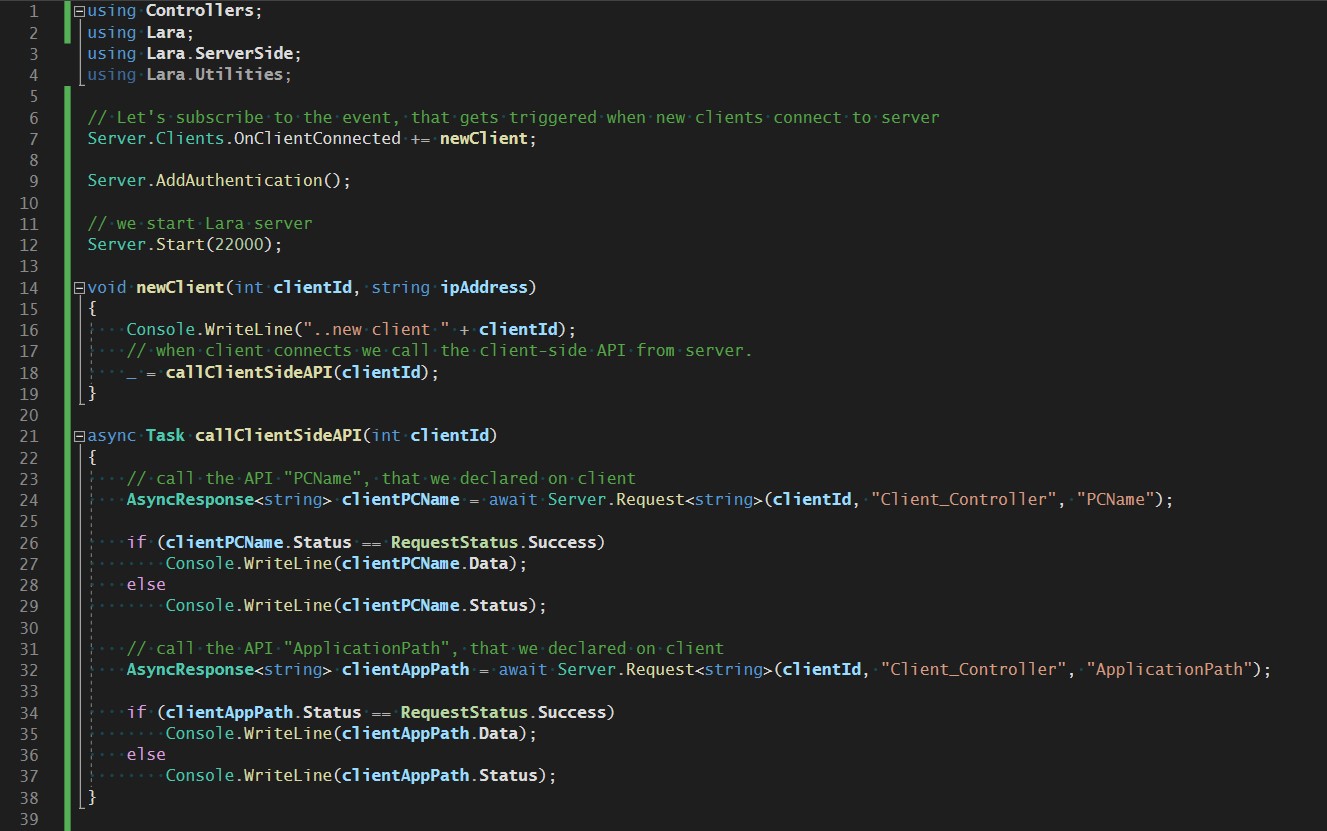
Console output:
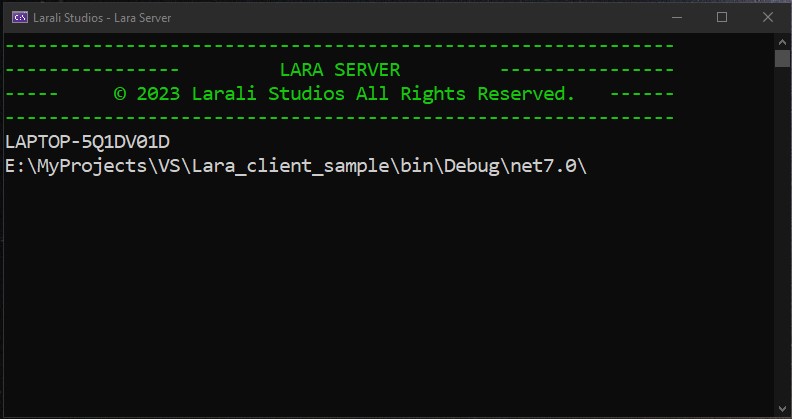
Back to authentication system
Go to Serialization system